The GestureInfo struct contains information about what the hand is doing.
For more information about the different gestures please look at https://developer.manomotion.com/supported-gestures/
Here are some examples of how you can access and use the gesture information.
You can access the gesture information from the HandInfos in ManoMotionManager like this:
void Update()
{
GestureInfo gestureInfo = ManoMotionManager.Instance.HandInfos[0].gestureInfo;
}
ManoGestureContinuous
This value is set when a gesture is performed over several frames.
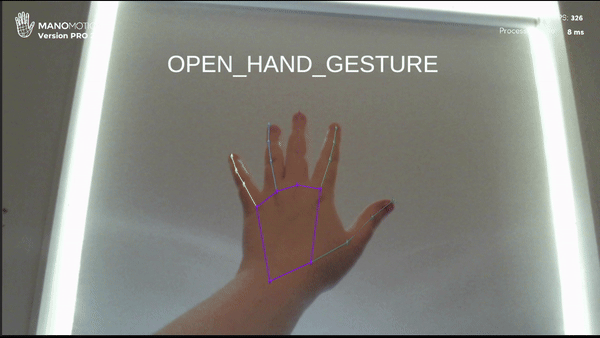
[SerializeField] TMP_Text continuousGestureText;
void ContinuousGestureExample(GestureInfo gestureInfo)
{
ManoGestureContinuous continuousGesture = gestureInfo.manoGestureContinuous;
continuousGestureText.text = continuousGesture.ToString();
}
ManoGestureTrigger
This value is set the first frame a gesture is performed.
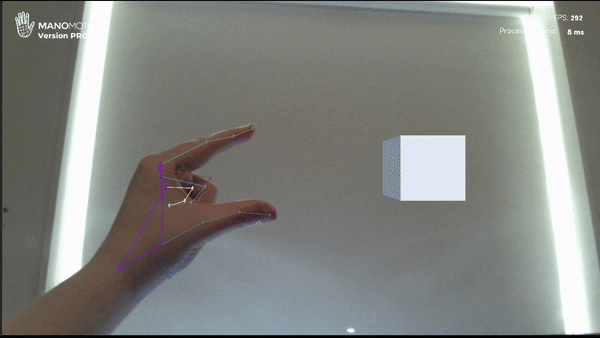
[SerializeField] GameObject toggleObject;
void GestureTriggerExample(GestureInfo gestureInfo)
{
ManoGestureTrigger gestureTrigger = gestureInfo.manoGestureTrigger;
if (gestureTrigger == ManoGestureTrigger.CLICK)
{
bool active = toggleObject.activeSelf;
toggleObject.SetActive(!active);
}
}
HandSide
Which side of the hand is facing the camera.
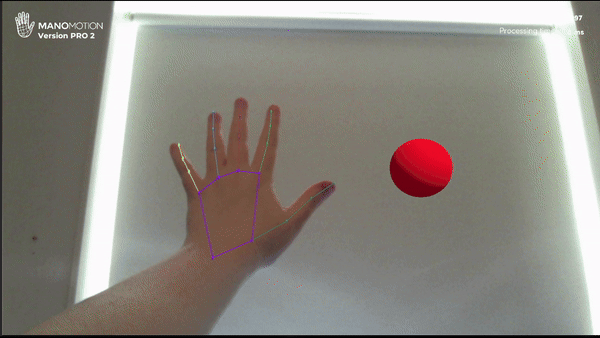
[SerializeField] MeshRenderer handSideRenderer;
[SerializeField] Material palmMaterial, backMaterial;
void HandSideExample(GestureInfo gestureInfo)
{
HandSide handSide = gestureInfo.handSide;
if (handSide == HandSide.Palmside)
{
handSideRenderer.material = palmMaterial;
}
else
{
handSideRenderer.material = backMaterial;
}
}